Java inheritance
The concept of inheritance is one of the most important concepts in object-oriented programming, because it irreversibly affects how Java code is written. Inheritance is a mechanism for creating a new class from an existing class by providing properties and methods.
Inheritance is the third paradigm (how to use techniques/mold) of object-oriented programming (the first being encapsulation, the second the class structure).
Inheritance can be single or multiple.
It represents the relationship: is a kind of
Example:
A car is a vehicle
A motorcycle is a vehicle
An airplane is a vehicle
While the member object represents the relationship: has a
A car has a motor
A motorcycle has wheels
An airplane has brakes
Inheritance is the definition of one class by extending the characteristics of another class, for example: We created a Vehicle class. Thus, the Aircraft, Car and Motorcycle classes were able to inherit the characteristics of Vehicle.
Inheritance is implemented by building derived classes.
The graph of inheritance is as follows:
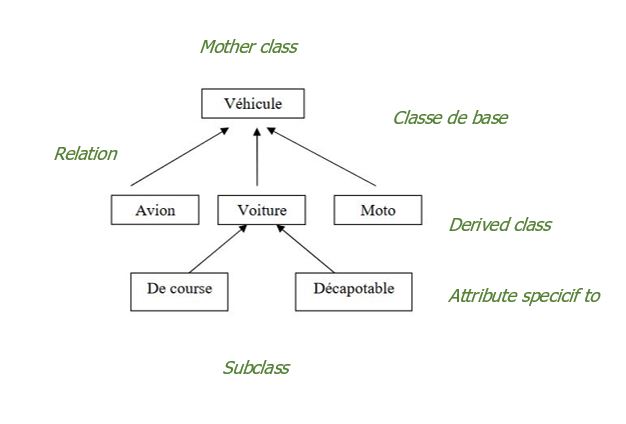
Tiering:
– the class from which one derives is called base class, superclass or mother class: Vehicle is the base class (upper class).
-classes obtained by derivation are called derived classes, subclasses or even daughter classes.
Airplane, Car and Motorcycle are derived classes (subclasses or daughter class).
Inheritance is one of the most powerful mechanisms of object-oriented programming.
Derived class:
A derived class models a particular case of the base class, and is enriched
more information.
The derived class has the following properties:
– contains the data members of the base class
– may possess new ones
– has (a priori) the methods of its base class
– may redefine (hide) some methods
– may have new methods
Derived class inherits members from base class
Mother-class superclass
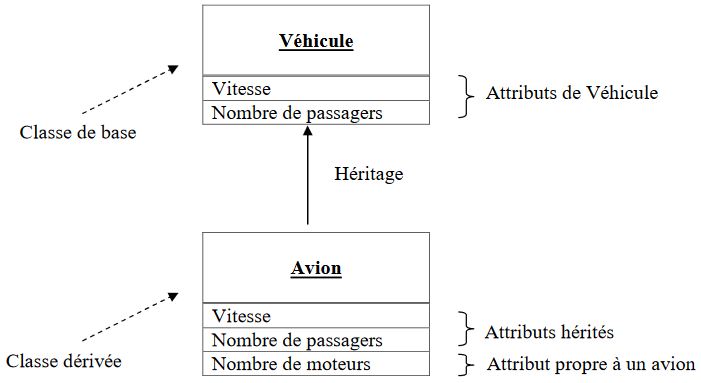
Subclass daughter class
Manufacturer:
– The derived class must support the construction of the base class.
To build an Airplane, you must first build a Vehicle;
The constructor of the base class (Vehicle) is therefore called before the constructor derived class (Airplane).
-1 The base class and the derived class have at least one public constructor, this is the general case:
Therefore, the constructor of the derived class must call the constructor of the available base class.
-2 The base class has no constructor:
The derived class may not explicitly call the base class constructor. If it does, it can only call the default constructor, since it is the only one available in this case in the base class.
-3 Derived class has any constructor:
In this case, the base class must have a public constructor with no arguments (default or explicit)
Access rights:
Access rights protect data and methods, and also perform encapsulation.
Access rights are granted to member functions, or global functions.
The protection unit is the class: all objects in the class have the same protection.
If the base class members are:
– Public or ‘nothing’: members of the derived class will have access to these members (Fields and methods)
– Private: members of the derived class will not have access to private members of the base class and have access only to functions that are members of the class (that is, only by the class)
–Protected: a member of the base class declared protected is accessible to its derived classes as well as classes of the same package
In Java, this mechanism is implemented using the extends keyword
Example:
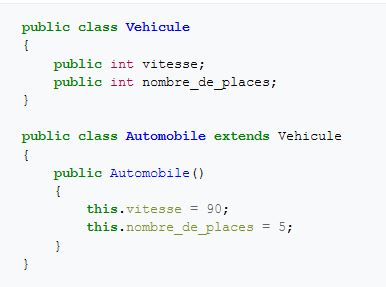
Just as the keyword this allows to refer to the current object, the keyword super allows to designate the superclass, that is, using the keyword super, it is possible to manipulate the member data and methods of the superclass.
In this example, the Automobile class inherits from the Vehicle class, which means that the speed and seat number attributes, although defined in the Vehicle class, are present in the Automobile class. The constructor defined in the Automobile class also initializes these attributes.
In Java, the inheritance mechanism allows you to define a class hierarchy that includes all classes. In the absence of explicitly specified inheritance, a class inherits implicitly from the Object class. This Object class is the root of the class hierarchy.
Reminder:
What is a constructor ?
A constructor is a particular function whose role is to create a new object from a class and initialize it
- Has the same name as the class that contains it
- A constructor does not return any values
- A constructor is always called using the new operator
The call to a constructor is made by the name of the class to be instantiated.
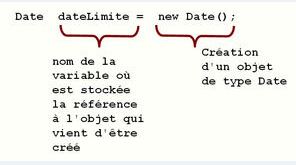

Constructor and inheritance
- If a constructor is defined in a class without starting with a constructor call, Java inserts a call from the default constructor of the super class.
- If a constructor of the derived class explicitly calls a constructor of the base class, this call must be the first constructor statement. He has to use the super keyword for that.
– When constructing the derived class, we must take into account the presence of the constructor in the base class and/or in the derived class.
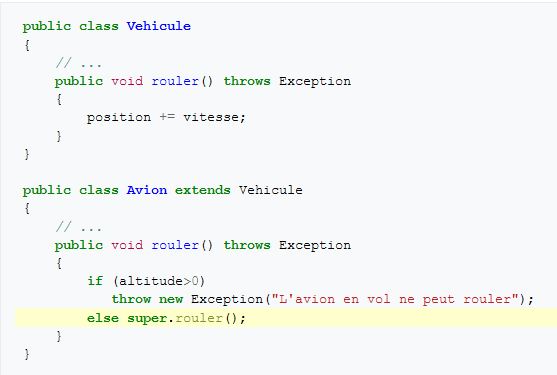