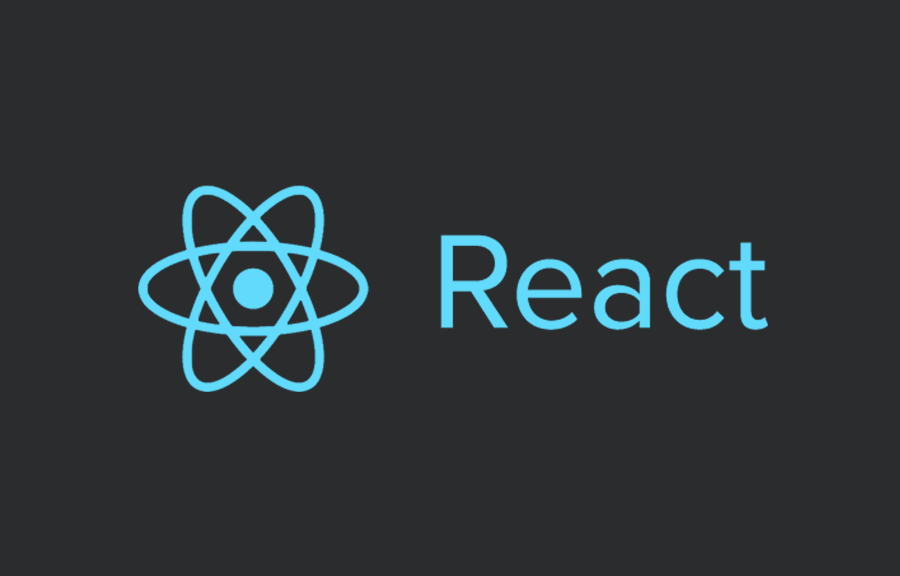
React JS
Developed by Facebook, React JS is an OpenSource Javascript library that allows the building of user interfaces (UI). It enables to create complicated UI from isolated little pieces of code called « component ». It is only concerned with what you can see on the front-end level.
Before React JS, programmers had to « build » UIs by hand. Now, with React JS, you just describe what you want and React will build the UI on your behalf in the web browser.
1/ Introduction
ReactJS has two extensions:
– JSX
– Virtual DOM
a) JSX
JSX is a ReactJS extension that make easier to alter the Document Object Model (DOM). The DOM is like a tree of how the web page is arranged.
b) Virtual DOM
When using JSX, ReactJS creates a Virtual DOM that is the copy of the web site’s DOM, in order to find which part of the true DOM needs to be modify when an event happens. This selective updating way makes it faster because only the part that needs to be modify is changed, not all the DOM.
2/ React Components
A React component is a JavaScript function that returns a little piece of a code that represents a part of a web page.
The functions are called in a certain order and put together to create the web page.
By doing this, the UI is splited in indepedent elements that can be used again later.
a) Functional components
Writing a JavaScript function is the easiest way to define a component:
function Welcome(props) {
return <h1>Hello, {props.name}</h1>;
}
This function is a valid React component because it accepts only one argument (props, which mean property) that contains datas and returns a React element.
b) Class component
This is also possible to write components as JS classes.
class Welcome extends React.Component {
render() {
return <h1>Hello, {this.props.name}</h1>;
}
}
Class components must have a function called render()
. The render function returns the JSX of the component.
3/ Producing the rendering
Elements can be defined by the user :
function Welcome(props) { return <h1>Hello, {props.name}</h1>;
}
const element = <Welcome name="CDA Team" />; // This constant is defined by the user !!
ReactDOM.render(
element,
document.getElementById('root')
);
When React runs into an element depicted a component defined by the user, it passes the JSX attributs and the children to this components as a unique object. This object is called « props ».
To resume :
– We call ReactDOM.render()
with the <Welcome name="CDA Team" />
element.
– React call the Welcome
component with {name: 'CDA Team'} as props.
– The Welcome
component return the element <h1>Hello, CDA Team</h1>
as a result.
– React DOM updates the DOM to match <h1>Hello, CDA Team</h1>
.
4/ Conclusion
ReactJS is a good solution to build complex UI with lots of components that are changing frequently over time, without having to write a lot of JS Code.
You can check Axel Teillier ‘s Article about ReactNative to go further.
https://www.freecodecamp.org
https://fr.reactjs.org
One thought on “React JS”