The <canvas> element
<canvas> is an HTML5 element that allows the creation and manipulation of graphical elements (paths, shapes, text, bitmap images) via a scripting language, most often JavaScript.
All modern browsers support it natively, and can render it using hardware acceleration.
To start, we add the following tag to the page, specifying the width and height attributes:
<canvas id="myCanvas" width="400" height="200"></canvas>
Drawing lines
Let’s draw a primitive C using two lines:
<script>
// find canvas element in DOM
var canvas = document.getElementById("myCanvas");
//context object provides drawing methods
var ctx = canvas.getContext("2d");
ctx.moveTo(100, 50); // start C at x=100, y=50
ctx.lineTo(50, 100); // middle of C is 50px behind, 50px below
ctx.lineTo(100, 150); // end of C is back at x=100, but y=150
ctx.lineWidth = 10; // set line width to 10px
ctx.strokeStyle = '#007bff'; // set line color to blue
ctx.stroke(); // apply stroke to canvas
</script>
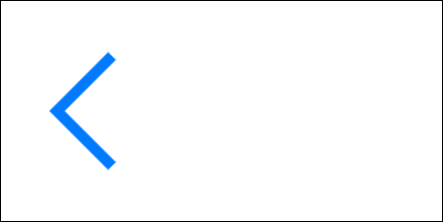
Drawing a shape
Now, let’s add a D using the context method arc. It accepts the following arguments:
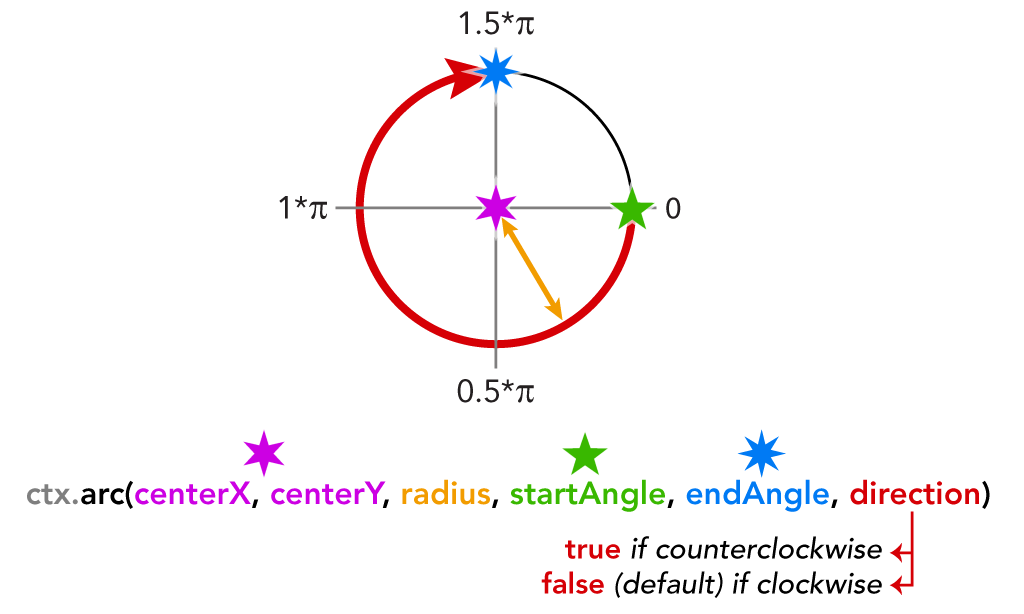
<script>
ctx.beginPath(); // new path
// arc: center | r | circle top | circle bottom | clockwise
ctx.arc(150, 100, 50, 1.5*Math.PI, 0.5*Math.PI);
ctx.closePath(); // close off the left part of the D
ctx.fillStyle = '#FFA800'; // solid orange fill
ctx.fill(); // apply fill to canvas
ctx.stroke(); // apply stroke to canvas
// stroke color is still #007bff, and width still 10px
</script>
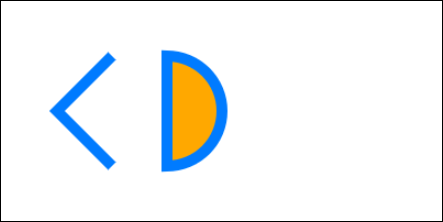
Drawing text
Next up, we’ll write an A using the context method fillText:
<script>
ctx.font = '150px Arial, sans-serif'; // text properties
ctx.textAlign = "center"; // horizontally centered on origin
ctx.textBaseline = "middle"; // vertically centered on origin
ctx.fillText('A', 270, 115); // write 'A', specifying origin
// fill stays '#FFA800', as before.
</script>
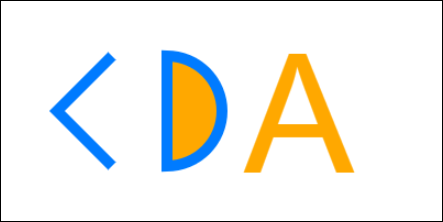
Manipulating <canvas> with JavaScript
Finally, let’s write a pulse function that makes the letter A grow and shrink cyclically.
<script>
var scale, angle = 0;
function pulse() {
angle += 0.05; // increment angle so that cosinus
// changes cyclically from -1 to 1
scale = Math.abs(Math.cos(angle)); // scale from 0 to 1
// clear right of canvas starting from x=210px (after 'D')
ctx.clearRect(210, 0, canvas.width, canvas.height);
ctx.setTransform(scale, // X scale, from 0 to 1
0, // vertical skewing
0, // horizontal skewing
scale, // Y scale, from 0 to 1
270-270*scale, // horizontal translation:
// Transform origin is 0,0 so translation must be 0 when scale
// is 1, and 270 when scale is 0
115-115*scale);// vertical translation
ctx.fillText('A', 270, 115); // apply transformed text;
requestAnimationFrame(pulse); // tell browser to call function
// again when ready to repaint.
}
pulse();
</script>
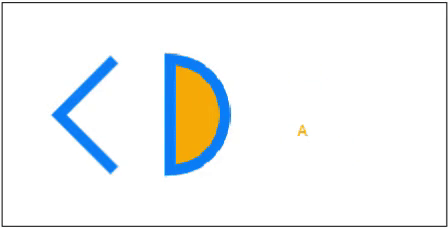
Final words
This brief introduction to <canvas> only scratches the surface of what it can do to help build complex applications and games. It is now mature and widely adopted across the web. With the decline of native apps and the rise of web apps, its importance can only increase in the years ahead.